El siguiente es un sencillo script de Python que se basa en web scraping y la API de OpenAI para recolectar y clasificar contenido de perfiles de TikTok.
Recopilación de Datos con Web Scraping
El primer paso para el análisis es recolectar los datos. En este caso, utilizando el módulo de requests de Python se obtiene el código HTML del perfil de TikTok. Posteriormente, se utiliza BeautifulSoup para analizarlo y extraer información relevante sobre los videos en el perfil. En particular, el título , el número de vistas y la URL.
URL Perfil: https://www.tiktok.com/@fahorro
Estructura HTML de un perfil de TikTok
La siguiente imagen muestra el código HTML del perfil en el recuadro naranja se observa la estructura de un bloque de video y podemos ver que los videos están en la clase class_='tiktok-x6y88p-Div... , con esta información se genera el script.
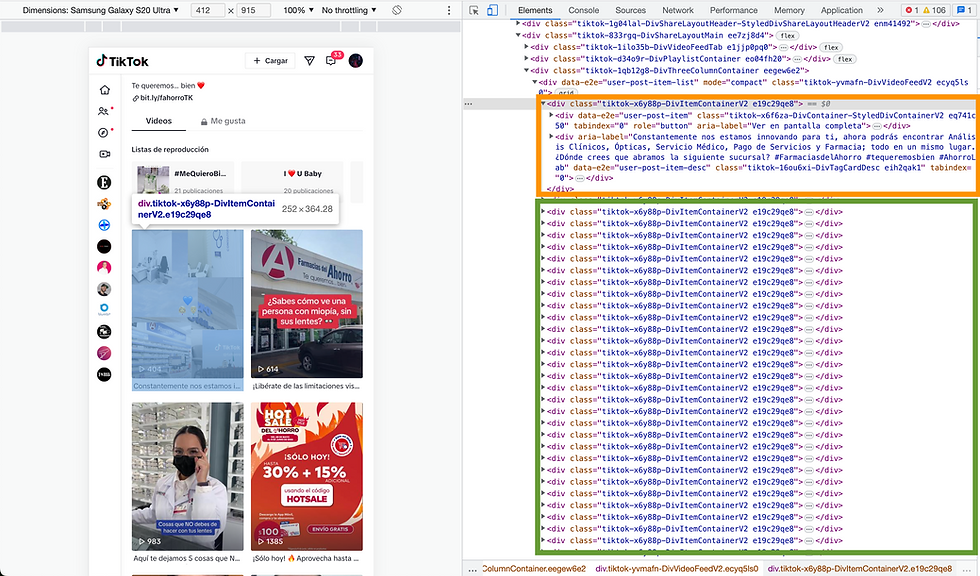
from bs4 import BeautifulSoup
import requests
import csv
#Perfil Tiktok
url = "https://www.tiktok.com/@fahorro"
#Solicitud HTTP
response = requests.get(url)
#Verificar si la solicitud fue exitosa
if response.status_code == 200:
#Obtener codigo html
html_doc = response.content
# analizar html con BS y html.parser
soup = BeautifulSoup(html_doc, 'html.parser')
# Encontrar las classes de los videos "tiktok-x6y88p-Di... divs = soup.find_all('div', class_='tiktok-x6y88p-DivItemContainerV2 e19c29qe8')
# Generar nombre de usario de la url
username = url.split('@')[1]
# guardar archivo csv con nombre de usuario
filename = f"{username}.csv"
# CSV
with open(filename, 'w', newline='') as file:
writer = csv.writer(file)
# Titulos
writer.writerow(["Video Title", "Views", "URL"])
for div in divs:
# Obtener Video Caption
video_caption = div.find('a', class_='tiktok-1wrhn5c-AMetaCaptionLine eih2qak0')['title']
# Obtener Video Views
video_views = div.find('strong', class_='video-count tiktok-dirst9-StrongVideoCount e148ts222').text
# Guardar CSV
writer.writerow([video_caption, video_views])
print("Video Caption: ", video_caption)
print("Video Views: ", video_views)
print("----------")
print("CSV file saved successfully.")
Categorización de Datos con OpenAI
Una vez recopilados los datos, cada video se debe clasificar en una serie de categorías o pilares de contenido basados en el título. Estos son:
Promociones y Ofertas
Muestra de Productos
Muestra de Servicios
Testimonios
Contenido Educativo
Contenido Inspiracional
Colaboración con Influencer/Celebridad
Contenido Generado por el Usuario
Responsabilidad Social Corporativa
Engagement.
Para lograr esto, se define una función de instrucciones que toma como parámetros una lista de mensajes, el promt y el titulo del video.
El promt, indica que el titulo del video se deberá clasificar de acuerdo a las categorías predefinidas y utilizando la función ChatCompletion de OpenAI, la API devuelve una respuesta que representa la categoría en que el video se clasificaría mejor y se añade como columna al archivo un archivo CSV.
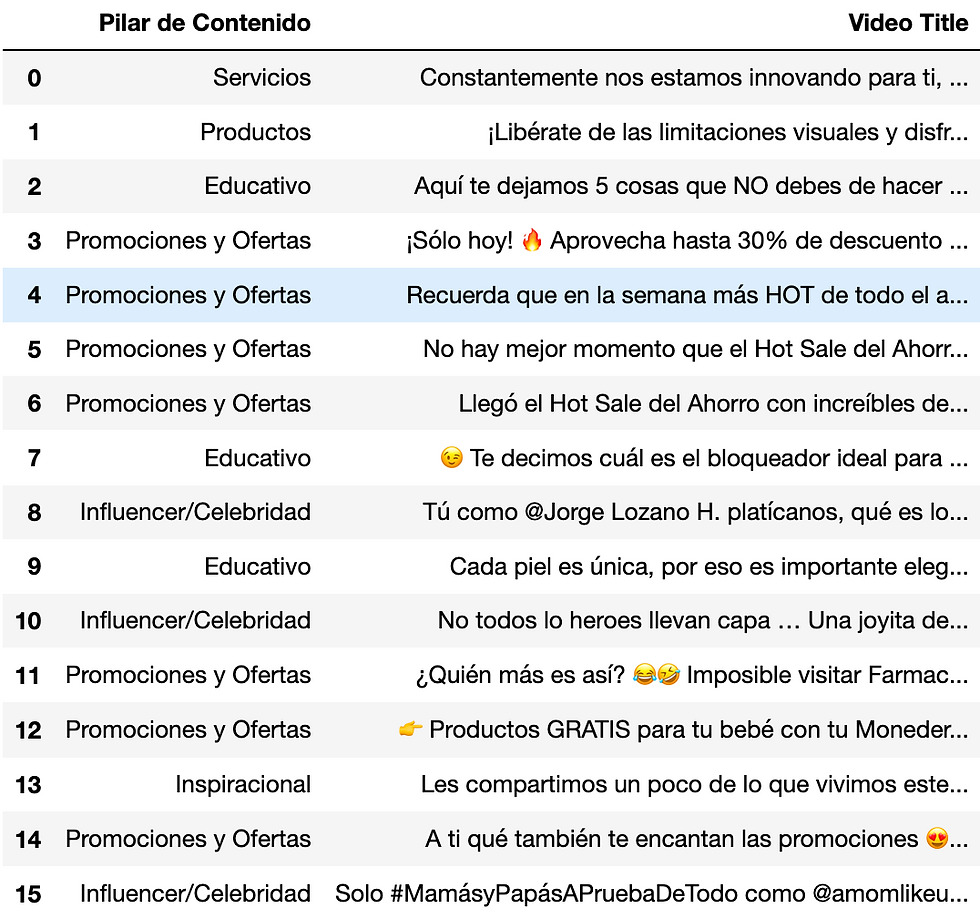
import os
import openai
from dotenv
import load_dotenv
import pandas as pd
load_dotenv()
#API KEY
openai.api_key = os.environ['OPENAI_API']
#Archivo CSV con videos
data = pd.read_csv('fahorro.csv')
#Definicion de funcion
def clasificacion(mensajes,
modelo="gpt-4",
temperatura=0,
max_tokens=500):
respuesta = openai.ChatCompletion.create(
model=modelo,
messages=mensajes,
temperature=temperatura,
max_tokens=max_tokens )
return respuesta.choices[0].message["content"]
#Definicion de instruccion
delimitador ='@@@@'
instruccion = f""" You will be provided with tiktok video data in spanish. The data will be in the form of a csv row. \ The structure of the csv is: Video Caption,Video Views,Video URL \ Each video data will be delimiter by {delimitador}. Your job is to categorize them accordingly. The categories are: < Promociones y Ofertas: The title includes advertising of discounts, special offers, and promotional deals > < Productos: The title refers to detailed demonstrations of a brand's products, which may include unboxings, feature highlights, or product comparisons > < Servicios: The title signifies an in-depth look at the services a brand offers, often demonstrating the service process or results > < Testimonial: The title indicates customer endorsements, featuring statements about their positive experiences with a brand's products or services > < Educativo: The title pertains to content designed to educate the audience, typically on a topic related to the brand's industry. This can include how-to guides, tutorials, and informational content > < Inspiracional: The title represents content intended to inspire or motivate the audience, such as stories of personal achievement or overcoming adversity > < Influencer/Celebridad: The title refers to content featuring influencers or celebrities endorsing or using a brand's products or services > < User-Generated Content: The title signifies content created by the brand's audience or customers, which can include product use, reviews, or participation in brand campaigns > < Responsabilidad Social: The title indicates content highlighting a brand's social, environmental, or philanthropic initiatives > < Engagement: The title pertains to videos designed to encourage user action, conversion, or interaction with the brand or its content > Your answer should be only the cateogry. """
#Lista de categorias vacia
categorias =[]
for index,row in data.iterrows():
videoRow = row['Video Title']
mensajes = [
{
'role' : 'system',
'content' : instruccion},
{
'role' : 'user',
'content' : f""" {delimitador} {videoRow} {delimitador} """}
]
analisis = clasificacion(mensajes)
categorias.append(analisis)
#Crear columna de Pilar de contenido en archivo csv
data['Pilar de Contenido'] = categorias
#Guardar dataframe en nuevo archivo csv data.to_csv('fahorroCategorias.csv', index=False)
Analisis Exploratorio de Data (DEA)
Utilizando librerías como Pandas, matplotlib y Plotly se puede observar que:
La mitad de los videos de este perfil se categorizan bajo el pilar de contenido de Productos, no obstante, no es el pilar que atrae la mayor cantidad de visitas, ocupando el quinto lugar entre diez categorías.
El contenido con mayor número de vistas es el de Responsabilidad Social, sin embargo es el pilar con menor cantidad de videos.
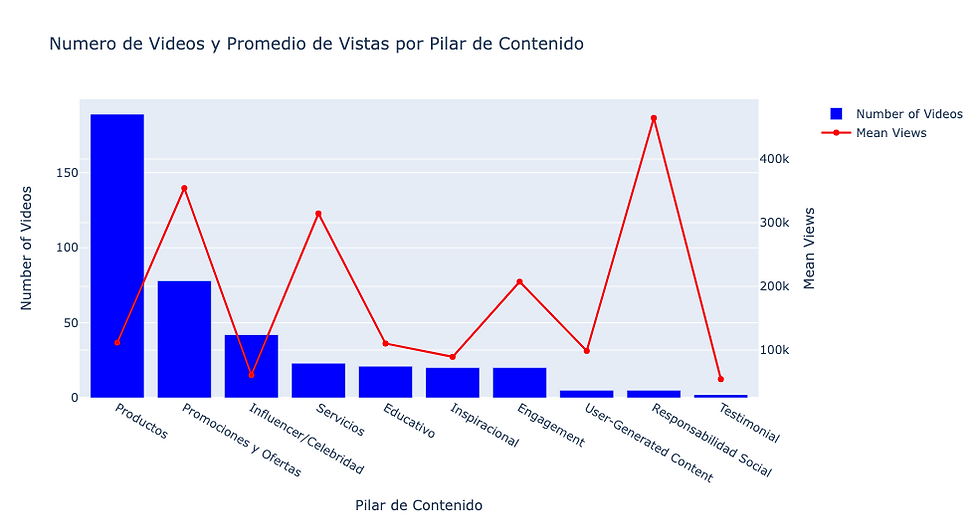
Nota: Este análisis no toma en cuenta si las visualizaciones de los videos son de origen orgánico o resultantes de publicidad paga.
Comments